반응형
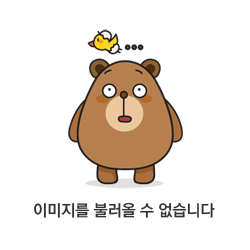
1. 화살표 함수란?
화살표 함수(Arrow Function)는 **ES6(ECMAScript 2015)**에서 도입된 함수 선언 방식으로, 기존의 function 키워드를 사용하는 방식보다 간결한 문법을 제공합니다. 또한, this의 바인딩 방식이 다르기 때문에 기존 함수와 차이점이 있습니다.
기존의 **함수 표현식(Function Expression)**과 비교해보면 다음과 같습니다.
기존 함수 표현식
const add = function(a, b) {
return a + b;
};
console.log(add(3, 5)); // 8
화살표 함수
const add = (a, b) => a + b;
console.log(add(3, 5)); // 8
✅ 차이점:
- function 키워드 생략
- 중괄호({})와 return 키워드 생략 가능 (한 줄로 작성 가능할 경우)
- 더 간결하고 가독성이 좋음
2. 화살표 함수의 기본 문법
2.1 일반적인 형태
const 함수이름 = (매개변수) => {
실행할 코드;
return 반환값;
};
- 매개변수가 1개일 경우 () 생략 가능
- 실행할 코드가 1줄이고 return 문을 포함하면 {}와 return 키워드 생략 가능
2.2 다양한 사용 예제
(1) 매개변수가 없는 경우
const greet = () => "Hello, World!";
console.log(greet()); // Hello, World!
(2) 매개변수가 하나인 경우
const square = x => x * x;
console.log(square(4)); // 16
(3) 여러 줄의 코드가 있는 경우
const multiply = (a, b) => {
console.log(`Multiplying ${a} and ${b}`);
return a * b;
};
console.log(multiply(3, 4)); // 12
(4) 객체를 반환하는 경우
객체를 반환할 때는 {}를 **소괄호(())**로 감싸야 합니다.
const getUser = (name, age) => ({ name, age });
console.log(getUser("John", 30));
// { name: "John", age: 30 }
3. 콜백 함수로의 활용
화살표 함수는 **콜백 함수(callback function)**로 자주 사용됩니다.
(1) 배열 map() 메서드 사용
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map(n => n * n);
console.log(squaredNumbers); // [1, 4, 9, 16, 25]
(2) filter() 메서드 사용
const evenNumbers = numbers.filter(n => n % 2 === 0);
console.log(evenNumbers); // [2, 4]
(3) reduce() 메서드 사용
const sum = numbers.reduce((acc, curr) => acc + curr, 0);
console.log(sum); // 15
4. 화살표 함수와 this 바인딩
화살표 함수는 자신만의 this를 가지지 않고, 상위 스코프의 this를 그대로 사용합니다.
4.1 일반 함수의 this 바인딩
const person = {
name: "Alice",
sayHello: function() {
console.log(`Hello, I am ${this.name}`);
}
};
person.sayHello(); // Hello, I am Alice
4.2 화살표 함수의 this 바인딩
const person = {
name: "Alice",
sayHello: () => {
console.log(`Hello, I am ${this.name}`);
}
};
person.sayHello(); // Hello, I am undefined
🔹 왜 undefined?
- 화살표 함수는 this를 현재 객체(person)에 바인딩하지 않음
- this는 전역 객체(window 또는 global)에서 가져옴
✅ 해결 방법: 일반 함수 표현식 사용
const person = {
name: "Alice",
sayHello: function() {
console.log(`Hello, I am ${this.name}`);
}
};
person.sayHello(); // Hello, I am Alice
5. 화살표 함수 사용 시 주의할 점
✅ 1) this가 필요한 경우 사용 X
const user = {
name: "Bob",
getName: () => this.name
};
console.log(user.getName()); // undefined
✅ 2) arguments 객체 사용 불가능
화살표 함수에는 arguments 객체가 존재하지 않습니다.
const func = () => console.log(arguments);
func(1, 2, 3); // Uncaught ReferenceError: arguments is not defined
✅ 해결 방법: rest 파라미터(...args) 사용
const func = (...args) => console.log(args);
func(1, 2, 3); // [1, 2, 3]
6. 결론: 언제 화살표 함수를 사용할까?
✅ 화살표 함수를 사용하기 적합한 경우
- 짧고 간결한 함수를 만들 때
- 콜백 함수 (map(), filter(), reduce(), setTimeout() 등) 사용 시
- this 바인딩이 필요 없는 함수 (ex: 일반적인 유틸리티 함수)
🚫 화살표 함수를 사용하지 말아야 하는 경우
- 객체의 메서드 (this를 사용해야 할 때)
- 생성자 함수 (new 키워드 사용 불가)
- arguments 객체가 필요한 경우
반응형
'IT > Javascript Basic' 카테고리의 다른 글
[javascript] 비동기 처리 개념 설명 (0) | 2025.02.16 |
---|---|
[javascript] 삼항 연산자 기본 개념 (0) | 2025.02.14 |
[javascript] 로컬 스토리지(Local Storage)란? (0) | 2025.02.09 |
[javascript] 자바스크립트 중급 문법 학습 자료 (1) | 2025.02.09 |
[javascript] 자바스크립트 전역변수 및 지역변수의 호출 및 반환 방법 (0) | 2025.02.09 |
자바스크립트 초급(기초) 문법 학습 자료 (0) | 2025.02.09 |
[javascript 초급 중급 고급] 자바스크립트 초급부터 고급까지 - 자바스크립트 커리큘럼 추천 (0) | 2025.02.08 |
[javascript, console.log] console.log와 console.error의 차이점 (0) | 2025.02.07 |
댓글